Recent Changes and Additions:
July 31, 2014: Added optional parameter &cancelparked to cancelallpending command.
|
Overview
Many traders use computer programs to place trades. To accommodate these traders,
Collective2 makes available a Signal Entry API. All calls to
this API are accomplished through HTTP, by having your
program call a URL.
Who can use it
Using the Signal Entry API is easy. Your software
needs to be able to access a Web URL. (It needs to be able
to 'GET' a Web page.) This is a trivial
matter in Perl, C++, or VB. If you need assistance with
this, please post a message on our Forums; I'm sure
you will be able to get help from one of our geeky members.
Note that you can manually test this interface by simply
typing a Web address into your browser. This will allow you
to see how the system works.
How to create the URL
Before you begin, you'll need to have created at least
one trading system on Collective2. You'll need also to know
the system id of the trading system for which you want to
place a trade. (Every system on Collective2 has an unique id
number associated with it. This is visible to the system
owners when they visit their "System Details" page
on Collective2.)
|
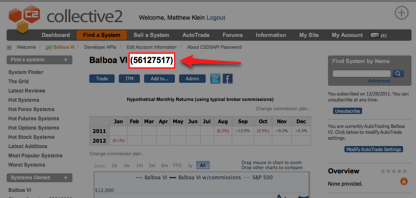 |
|
|
|
|
Above: you will find
your system's id on the system details page. The
number is not meant to be secret, but it is
displayed only when you are logged in as the
system's owner. |
|
|
|
|
|
The strategy for using the Web interface is as follows.
You will code a web address (URL) which contains all the
information necessary to place a trade. Unless you specify
otherwise, the trade will become valid immediately, and also
will be emailed (or Instant Trade Messengered) to
subscribers immediately.
The URL is in the following format:
http://www.collective2.com/cgi-perl/signal.mpl?[PARAMETERS]
Placing an order
The required parameters are:
Parameter |
Value or Example |
What it Means |
Comments |
cmd= |
signal |
|
|
systemid= |
123456 |
The system ID displayed as above. |
|
pw= |
loginPassword or (preferred) C2 Data Services password |
case sensitive |
action= |
BTO |
Buy To Open |
open a long position |
|
SSHORT |
Sell Short |
used for stocks |
|
STO |
Sell To Open |
used for non-stocks |
|
BTC |
Buy To Close |
close a short position |
|
STC |
Sell To Close |
close a long position |
quant= |
100 |
Number of shares or contracts
Note: for forex, use the number of minilots (10,000 cus). Example: submitting "Buy 2" EURUSD will effectively buy 20,000 Euros. |
dollars= 3500
|
Optional. May be used instead of quant.
For stocks only, you may submit a dollar value, which will be translated into a number of shares. Price used may be delayed. If order submitted when market is closed, the last trade price from the previous day will be used to calculate shares, not the next day opening price. |
instrument= |
stock |
Note that ETFs like
QQQ and DIA are traded on a stock exchange and thus
are considered stocks. |
|
option |
|
|
|
future |
|
|
|
forex |
|
|
symbol= |
IBM |
|
You can find C2 Symbols here. |
limit= |
35.06 |
|
Only use if this is a limit order. |
stop= |
20.10 |
|
Only use if this is
a stop order. For market orders, do not use either
stop or limit parameters. |
duration= |
DAY |
Day Order |
|
|
GTC |
Good Til Cancel |
|
The table above outlines the basic URL format. Here are
two examples to demonstrate.
 |
You want to buy 1200 shares of IBM at the market. |
|
|
|
Your program calls the following URL
(all on one line): |
|
|
http://www.collective2.
com/cgi-perl/signal.
mpl?cmd=signal&
systemid=1234&pw=abcd&instrument=stock&action=BTO&quant=1200&
symbol=IBM&duration=DAY |
|
|
|
 |
You want to buy 5 contracts of the December 2011
E-Mini S&P at limit 1120 or better, good til
cancel. (For help with futures symbols, see this page.) |
|
|
|
Your program calls the following URL
(all on one line): |
|
|
http://www.collective2
.com/cgi-perl/signal.
mpl?cmd=signal&
systemid=1234&pw=abcd&instrument=future&action=BTO&quant=5&
symbol=@ESZ1&limit=1120&duration=GTC |
|
After you or your program calls the URL, the Web site
will respond either with an error message, or with data
similar to the following:
<collective2>
<signalid>10344682</signalid>
<comments>Signal 10344682 accepted for immediate
processing.</comments>
</collective2>
This pseudo-XML text is designed so that you can more easily extract
the unique signal ID which is assigned to your request.
Every trading signal on Collective2 is given an unique
signal id.
Knowing the signal id of the trade you enter is useful
for more complex trades, like conditionals and OCA orders. In
addition, it is required when you want to cancel an order.
Using your own signalids
Every order on Collective2 has an unique signal id. The
easiest way to use the Signal Entry API is to place an order
using the ?cmd=signal parameter, as described above, and
then allow C2 to assign your signalid. Your software can
read the signalid sent back in the <signalid> node
(see example above). This signalid can be used for order
cancellation, creating conditionals (described below), and
creating OCA groups (described below).
In the example above, C2 assigned your order a signalid.
However it may be easier to use your own signalids when
creating an order. There are a few important things to note
about this. First, if you create your own signalid, you must
guarantee its uniqueness within your own trading system,
across eternity. Once you specify a signalid, you must not
use it again -- even years later -- and even if the original
order in which the signalid appeared has been
canceled, expired, etc.
To specify your own signalid, simply submit your signal id
numbers along with the order. For example:
http://www.collective2 .com/cgi-perl/signal. mpl?cmd=signal&
systemid=1234&pw=abcd&instrument=future&action=BTO&quant=5&
symbol=@ESZ1&duration=GTC&signalid=100
Thereafter you can use your own signal ids to cancel
orders and build conditionals.
If you specify a signalid, it must be a positive integer
that is greater than zero and less than or equal to
4294967295.
(Note that you are not required to submit your own
signalid. If you do not submit one, the server will generate
one for you.)
Conditional Orders
A conditional order is an order that does not become
valid until a preceding order is filled. This is useful, for
example, if you want to set a good til cancel stop loss, but
only after an initial order is filled first.
To place a conditional order, add the following parameter
to your URL:
&conditionalupon=100
Example:
http://www.collective2 .com/cgi-perl/signal. mpl?cmd=signal&
systemid=1234&pw=abcd&instrument=future&action=STC&quant=5&
symbol=@ESZ1&limit=1500&duration=GTC&conditionalupon=100
In the example above, you are saying, "Place the
following Sell To Close Limit Order... but only after
SignalID=100 has been executed. Until signalid has been
filled, this order should not be placed."
Reversal Orders (the hard way)
If you are long an instrument, and you want to go short
(or vice-versa) that is called a reversal. In order to
facilitate AutoTrading your C2 system, C2 insists that
reversal be accomplished in two steps: first you must send
an order to close the open position. Next you must send an
order to open the new position.
Example 1: You are long 100 shares of IBM. You
want to reverse at the market, so that you end up short at
the market. You must therefore issue two commands in
sequence:
STC 100 IBM @ MKT
STO 100 IBM @ MKT
Example 2: Your are short 2,000 EUR/USD, which is
trading at 1.1234. You want to reverse your position and go
long if the EUR/USD falls to 1.000.
This is best accomplished as a conditional order like
this:
BTC 2,000 EUR/USD at limit 1.000
conditional order: BTO 2,000 EUR/USD at MKT (when
order above fills)
Reversal Orders (the easy way)
If you would rather have C2 handle the complexities or
reversal orders, you can use our one-step reversal command.
The most simple format is like this:
?cmd=reverse&symbol=EURUSD ...
The command above will simply flip your current position
from long to short, at the market price, immediately. You
can specify additional parameters if you like. The most
useful of them is a "triggerprice" at which the
reversal should take place:
?cmd=reverse&symbol=EURUSD&triggerprice=1.000
...
Here are the reversal-related parameters you can use:
cmd=reverse (required)
symbol=SYMBOL (required)
triggerprice=price (optional)
duration=DAY/GTC (optional; will be GTC unless
specified)
quant=new opening quant (optional; use only if
you want your final position to be a quantity different
than your prior quantity; if not specified, you will go
from long to short, or short to long, using the same
quantity of position before the reversal)
OCA Groups
You can specify that a group of trades act as a
One-Cancels-Another (OCA) group. The definition of an OCA
group is this: as soon as the first order within the group
is filled, the remaining orders within the group are
cancelled.
To make an oca group, you need to specify the ocagroupid
with each signal you enter. The ocagroupid is an unique
number. You can either specify your own ocagroupid, or can
request one from the C2 server.
To request an OCA id number, send the following command:
?cmd=requestocaid
You will receive the following response:
<collective2>
<ocaid>17195788</ocaid>
<status>You may use the ocaid above when adding new signals.</status>
</collective2>
You can then use this OCA id when placing trades. Just
add the OCA id to each trade signal URL, as in the following
example:
http://www.collective2 .com/cgi-perl/signal. mpl?cmd=signal&
systemid=1234&pw=abcd&instrument=future&action=BTO&quant=5&
symbol=@ESZ1&duration=GTC&ocaid=12345
Alternately, you can simply manufacture your own unique
never-to-be-used-again ocaid.
Obviously, for an OCA group to function properly, more
than one signal will need to be identified with the OCA id.
Once a group of 2 or more orders are entered using the same
OCA id, the first trade that gets executed will immediately
cancel the other trades within the OCA group.
Before this latest feature, you were required to send (1)
your entry order, (2) your stop loss, and (3) your profit
target as three separate orders. If you wanted to do
everything perfectly, you were required to make your stop
and profit-target conditional upon the entry order being
filled, and also to make the stop and profit target a single
OCA group.
That was a lot of API drudgery for a common task. So we
developed an all-in-one order entry.
Essentially, you enter your order as shown above, but you
can tack on one or both of the following:
&stoploss=xxx
&profittarget=yyy
If you enter both a stoploss and a profittarget, the two
exit orders will automatically become a One-Cancels-Another
(OCA) group. That way, if you exit a trade at the profit
target (for example), your stoploss order won't be left
lying aroun.
Here's an example:
http://www.collective2 .com/cgi-perl/signal. mpl?cmd=signal&
systemid=1234&pw=abcd&instrument=future&action=BTO&quant=5&
symbol=@ESU6&limit=1000&duration=GTC&stoploss=900.50&profittarget=1200
Update:
November 13, 2007: &forcenooca=1
When you create an order with a stop loss and
profit target all-in-one, you are creating one parent order
(the entry order) and two conditional (children) orders.
(The children are the stop loss and profit target.)
As a convenience, C2 will automatically make the two
children a One-Cancels-Another (OCA) group. This means that
if either child is filled or cancelled, so too is the other
child.
However, there are cases where this convenience is most
decidedly not a convenience. In particular, in cases
where a system will often cancel and then replace a
stop-loss or profit target, it is annoying that this action
will affect the other unrelated child order.
So you can specify &forcenooca=1 whenever
you submit a signal. This will prevent an OCA group from
being created automatically. While OCAs are only created
automatically in cases where both profittarget and
stoploss are included in one single API submission, there
is no harm in including the &forcenooca=1 in
all cases. It will simply be ignored in instances where it
is not applicable.
Advanced Topics
Relative Orders
C2 allows you to enter trade signals without knowing the
specific price of the instrument at the time you place the
orders. For example, you can specify that an order be placed
after the market opens, based on whatever the opening price
turns out to be. In this example, your order would look
something like "Sell To Open at OPENING PRICE -3
Stop"). In addition, you can set your profit targets
and stop losses based on whatever your initial fill price
turns out to be. So, for example, your order would look
something like this: BTO 1 IBM @ market, profit target=ENTRY
PRICE + 5, stop loss=ENTRY PRICE -2.
To learn more about "relative orders" such as
these, please see this
page.
Relative orders are also available through the API. The
URLs needed to encode them can be somewhat complex, so this
feature is meant for advanced users. This feature can best
be shown through an example:
Let's go through the example, piece by piece. First,
obviously, we provide system credentials (system id number
and password). Next we specify the opening portion of the
order: we will Buy To Open 1 electronic Soybeal Meal, at the
upcoming market open, at a limit price that is based on
whatever the market opens at.
Note that we do not know at what price the SM market will
open. But we can specify that our entry order will be at the
market opening price plus 66 points (or, O+66). A human
being would be tempted to write: O+66 but unfortunately web
services will puke at the "+" plus sign. We need
to "encode" all plus signas as the following
string: %2B ... in other words the phrase:
limit=O+66
becomes
limit=O%2B66
No such similar encoding is needed for minus signs.
Now let's continue reviewing the example above.
We are specifying that we want to enter the SM market at
a limit price of O+66. We could stop there, if we wanted,
but let's imagine that we also want to specify a profit
target and stop loss in the same command. Of course, our
stop loss and profit target will be based on the actual
trade price we receive in the opening order... which we
don't know yet. But that is not problem. We can represent
the opening trade price with a T.
So the last part of our string says:
Set a good-til-cancel stop loss and profit target as
follows (these are good-til-cancels because of the phrase
"&targetTIF=GTC"):
profittarget=opening trade price + 55
stoploss=opening trade price - 4
Whenever you use "relative orders" you can
specify one of three relative order types:
O --> represents the session opening price (be
careful of electronic contracts, as the opening of the
session is often the "night" before the main
trading day)
T --> represents the fill price of the opening
portion of the trade. This implies you can't use T+ or T-
orders for BTO or STO, since no open has been executed.
Q --> represents the real-time quote-feed price of
the instrument at the moment the order is released for
processing.
Keep in mind that you can add &parkuntildatetime
parameters to your opening order, which will allow you to
specify an order in advance of the time you want it
released. &parkuntildatetime is explained further below.
Delaying Signal Processing
As you can see, trading signals need to be entered one at a
time. Given this fact, you may wish to delay the processing of
groups of signals until the entire group is entered. Why would this be?
Well, for example, you may not want to confuse your
subscribers by sending them two trading signal emails in
rapid-fire succession (which is what will happen by default,
when you enter one signal after another.) Or, more
important, you may not want your first trading signal to be
"accidentally" traded until you are sure that your conditional order is in
place.
Solving this is simple. You can enter an optional
parameter when you construct your trading signal URL. It is delay=X, where X is the number of seconds you want to delay
a signal before processing it.
To illustrate, let's modify our second example URL from above.
Let's say you want to enter an order to buy 5 contracts of
the E-Mini S&P at limit 1120, but you don't want the
signal to be immediately traded. Also, you don't want the
signal to be immediately emailed to your subscribers,
because you would prefer to wait until you have entered
other signals first. Then, once all of your signals are
entered, you will send one email to each subscriber, containing
the batch of signals.
Here is the modified example URL, incorporating this
delay parameter:
|
|
 |
You want to buy 5 contracts of the
E-Mini S&P at limit 1120 or better, good til
cancel... but delay the email to subscribers (and
the trade itself) until 3 minutes from now. |
|
|
|
Your program calls the following URL
(all on one line): |
|
|
http://www.collective2
.com/cgi-perl/
signal.
mpl?cmd=signal&
systemid=1234&pw=abcd&instrument=future&action=BTO&quant=5&
symbol=@ESZ1&duration=GTC&delay=180 |
|
Note that 180 seconds is three minutes.
Delays and Emails To Subscribers
If you don't specify any delay, then trading signal
emails will be delivered immediately to your subscribers, and
trades themselves will be processed immediately (if the market
is open. Otherwise, the trade will be processed
when the market opens.)
On the other hand, if you specify a delay parameter, then
a signal will not be emailed immediately.
However, as soon as the first delay expires, then all
pending signals will be emailed to subscribers, all at once.
This is true, even if you have set individual delays for
subsequent trading signals.
This is not exactly obvious or intuitive, so let me try
to explain it in programming terms. Imagine there is a
routine called "sendAllPendingEmailsToSubscribers."
Normally, this routine gets called as soon as one trading
signal is entered. However, if you specify a delay=
parameter, then this routine is not called right away, but
is instead scheduled for a time in the future.
However, once the routine is executed, it emails all
trading signals which have not been sent yet. It doesn't
matter what individual delays you specified as you entered
each of these trading signals. The delay= parameter,
then, specifies the delay for scheduling the
sendAllPendingEmailsToSubscribers() routine. And that
routine simply packages up all pending trading signals,
creates an email containing them, and sends it to your
subscribers; it doesn't bother with worrying about delays or
schedules.
The point is that you should anticipate in advance of
entering your first trading signal when you will want all
subsequent signals emailed to subscribers. When you enter
your first signal in a given batch, specify the appropriate
delay. This will keep the sendAllPendingEmailsToSubscribers()
routine from being called until this delay has elapsed.
Thereafter, you must keep specifying a delay as you enter
more signals - to prevent the
sendAllPendingEmailsToSubscribers() routine from being
called until you are ready to have your emails sent to
subscribers. Your emails to subscribers will be sent at the
earlier of the following:
(1) When your earliest delay time expires
or
(2) The first time you specify a trading signal with no
delay
Canceling Orders
To cancel an order, simply issue a command like this:
http://www.collective2 .com/cgi-perl/signal. mpl
?cmd=cancel&signalid=XXX&systemid=123&pw=abcd
You can issue a blanket cancellation of all pending
orders for a trading system like this:
http://www.collective2 .com/cgi-perl/signal. mpl
?cmd=cancelallpending&systemid=123&pw=abcd
Add an optional &cancelparked=1 parameter to the above, to also cancel any unexecuted orders that have been parked.
Flushing signals
You can instruct the system to immediately process all
pending trade signals, despite any delays you have specified
earlier, by using the following command:
http://www.collective2 .com/cgi-perl/signal. mpl
?cmd=flushpendingsignals&systemid=123&pw=abcd
This overrides the earlier delays and insists that orders
get processed as soon as possible.
Time-based orders
You can schedule an order to become valid at a certain
time. Time is specified as the UNIX epoch time, that is, the
number of seconds since the UNIX epoch. This number is
available as function time within perl and is also available
through other programming languages. Use it like this:
http://www.collective2 .com/cgi-perl/signal. mpl?cmd=signal&
systemid=1234&pw=abcd&instrument=future&action=BTO&quant=5&
symbol=@ESZ1&duration=GTC&parkuntil=TIMEINSECS
You can also specify the time you want the order to
automatically cancel (if not filled). This is also specified
in UNIX time, like so:
http://www.collective2 .com/cgi-perl/signal. mpl?cmd=signal&
systemid=1234&pw=abcd&instrument=future&action=BTO&quant=5&
symbol=@ESZ1&duration=GTC&cancelsat=TIMEINSECS
Or, alternately, if you do not want to specify a clock
time at which the order cancels, you can instead specify a
"relative time" at which the order cancels. This
is number of seconds after the order has been submitted that
you wish the order to automatically cancel. For example, if
you want to make an order cancel automatically (if not
filled) 10 minutes after submitting it:
http://www.collective2 .com/cgi-perl/signal. mpl?cmd=signal&
systemid=1234&pw=abcd&instrument=future&action=BTO&quant=5&
symbol=@ESZ1&duration=GTC&cancelsatrelative=600
No need for date calculations: use
parkuntildatetime
Finally, if you don't want to deal with Unix
timestamps, you can park orders until a specific date and
time using parkuntildatetime=YYYYMMDDhhmmss
Example: You want to park an order until June 18, 2006 at
09:31:15 in the morning:
http://www.collective2 .com/cgi-perl/signal. mpl?cmd=signal&
systemid=1234&pw=abcd&instrument=future&action=BTO&quant=5&
symbol=@ESZ1&duration=GTC&parkuntildatetime=20060618093115
Note that all times are Eastern U.S. (New York City)
and use the 24-hr clock (4 pm is 16:00).
Closing all positions
You can instruct the system to close all
open positions. This will issue a set of closing orders, at
market, for any position you have open. You might want to
issue this at the end of the trading day, to make sure you
are flat before the markets close. If you have no positions
open, the command will be ignored:
http://www.collective2 .com/cgi-perl/signal.
mpl
?cmd=closeallpositions&systemid=123&pw=abcd
Changing stop and limits (the xreplace command)
C2 doesn't support automatic trailing stops (or trailing profit targets). This means you'll need to issue your stop loss, and then, as time passes, do a cancel-and-replace (xreplace) on it in order to change the trigger price. Let's look at an example of how to do this.
First, you place your original order:
http://www.collective2.com/cgi-perl/signal.mpl?cmd=signal&systemid=12345
&pw=mypassword&instrument=forex&action=BTO&quant=1&symbol=USDJPY
&duration=GTC&stoploss=101.90
Notice that I'm placing an "all-in-one" order: that is, an entry to go long at the market, plus my original stop loss (set at 101.90).
C2 responds with some ugly XML:
<collective2>
<ack>Signal Received</ack>
<status>OK</status>
<signalid>35584023</signalid>
<comments>Signal 35584023 accepted for immediate processing.</comments>
<oca></oca>
<delay></delay>
<stoplosssignalid>35584025</stoplosssignalid>
</collective2>
You'll want to parse out the <stoplosssignalid> tag, because you'll need it when it's time to change your stop loss.
How do we do it? Well, let's imagine you want to modify your stop loss to 101.80. You would:
http://www.collective2.com/cgi-perl/signal.mpl?cmd=signal&systemid=12345
&pw=mypassword&instrument=forex&action=STC&quant=1&symbol=eurusd
&duration=GTC&xreplace=35584025&stop=101.80
You can see that basically, I'm entering a new STC @ stop 101.80 order... but I'm specifying to first cancel signalid 35584025 (by using the xreplace=35584025 parameter).
C2 responds:
<collective2>
<ack>Signal Received</ack>
<status>OK</status>
<signalid>35584086</signalid>
Signal 35584086 accepted for immediate processing.</comments>
<oca></oca>
<delay></delay>
</collective2>
Requesting Buying Power
Some trading systems may vary the quantity
of the trades they place, or decide to take certain actions
such as canceling pending orders, depending on the amount of
"buying power" they have left. When your Buying
Power goes down to zero, Collective2 will issue a margin
call, and may begin to close any of your open in any order
it deems appropriate.
To request information about a particular
trading system's buying power, do this:
http://www.collective2 .com/cgi-perl/signal.
mpl
?cmd=getbuypower&systemid=123&pw=abcd
The response you receive back will look like
this:
<collective2>
<status>OK</status>
<calctime>1136058468</calctime>
<buypower>68300.00</buypower>
</collective2>
Buying power is recalculated every ten
minutes, or when a trade is opened or closed. The calctime
in the XML above is the Unix timestamp (seconds since the
beginning of the Unix epoch) when the buying power was
last calculated. In other words, there is no point in
requesting buying power more frequently than once every
ten minutes (or after a trade is executed).
The command called signalstatus
allows you to request the up-to-date status of a C2 order.
In other words, if you know a signalid, you can find
out whether a signal has been filled on C2's Hypothetical
Fill Engine, and at what price. You may also learn that the
signal has been canceled or has expired.
The requestor needs either to be the owner of the system in
question, or a subscriber to the system. You identify
yourself by passing the usual pw=c2password
parameter, but also -- and this part is new -- you must pass
the email address you use to login to C2. This last
parameter is necessary because this particular command does
not require you to specify which systemid you are
requesting information for. All that is necessary is the signalid.
Here's an example of how to call the command:
The response might look like this:
<collective2>
<signal>
<signalid>20919494</signalid>
<systemname>Velocity Forex System</systemname>
<postedwhen>2006-05-19 15:34:50:000</postedwhen>
<emailedwhen>2006-05-19 15:45:28:000</emailedwhen>
<killedwhen>0</killedwhen>
<tradedwhen>2006-05-19 22:08:53:000</tradedwhen>
<tradprice>20.87</tradeprice>
</signal>
</collective2>
Note that all times are Eastern U.S. time. A time field of
zero means it never happened (i.e. if "killedwhen"
is zero, then the signal never was killed/canceled).
Of course, for the command to be useful you must know the
signalid. Remember that you can specify your own signalids
when placing orders, or you can extract a C2-assigned
signalid if you do not use your own numbering scheme.
This command should help developers create order entry and
order-tracking applications. Keep in mind that this command
is not designed for AutoTrading use. It's a light-weight
command that simply reports order status on C2. It does not
determine whether a user should place a trade, or at what
quantities.
For AutoTrade development, be sure you look at the C2ATI
instead of the Trade Placement API (see below).
Update:
June 4, 2007
To answer a user request, we now allow your
API call for signalstatus to request more detailed
information. There are two new parameters you can use with
signalstatus. You can choose to use either, both, or
neither. Omitting both parameters means the data returned
will be exactly as above; this allows older software to
remain compatible.
The new parameters are:
&showrelated=[children|parent]
and
&showdetails=1
The first parameter specifies whether you
want C2 to return information about related signals. If you
specify &showrelated=children, then C2 will include
information about all orders that are conditional upon the
specified order. This is useful if you know an entry order's
signal id, but want to request information about related
stop-loss or profit-target orders.
The &showdetails=1 parameter tells C2
that you want C2 to return extended information about each
signal. This includes the buy/sell information, time in
force (TIF), stop, limit, quantity, and symbol.
An example will help demonstrate.
You call:
C2 responds:
<collective2>
<signal>
<signalid>26618011</signalid>
<systemname>Absolute Returns</systemname>
<postedwhen>2007-06-04 13:04:32:000</postedwhen>
<emailedwhen>2007-06-04 13:04:40:000</emailedwhen>
<killedwhen>0</killedwhen>
<expiredwhen>2007-06-04 16:18:09:000</expiredwhen>
<tradedwhen>0</tradedwhen>
<tradeprice>0</tradeprice>
<action>BTO</action>
<quant>100</quant>
<symbol>AAPL</symbol>
<limit>10.25</limit>
<stop>0</stop>
<market>0</market>
<tif>DAY</tif>
<ocagroupid></ocagroupid>
<child>
<signal>
<signalid>26618014</signalid>
<systemname>Absolute Returns</systemname>
<postedwhen>2007-06-04 13:04:32:000</postedwhen>
<emailedwhen>2007-06-04 13:04:40:000</emailedwhen>
<killedwhen>2007-06-04 18:53:25:000</killedwhen>
<expiredwhen>0</expiredwhen>
<tradedwhen>0</tradedwhen>
<tradeprice>0</tradeprice>
<action>STC</action>
<quant>100</quant>
<symbol>AAPL</symbol>
<limit>99.99</limit>
<stop>0</stop>
<market>0</market>
<tif>GTC</tif>
<ocagroupid></ocagroupid>
</signal>
</child>
<child>
<signal>
<signalid>26618016</signalid>
<systemname>Absolute Returns</systemname>
<postedwhen>2007-06-04 13:04:32:000</postedwhen>
<emailedwhen>2007-06-04 13:04:40:000</emailedwhen>
<killedwhen>2007-06-04 18:53:25:000</killedwhen>
<expiredwhen>0</expiredwhen>
<tradedwhen>0</tradedwhen>
<tradeprice>0</tradeprice>
<action>STC</action>
<quant>100</quant>
<symbol>AAPL</symbol>
<limit>0</limit>
<stop>7.5</stop>
<market>0</market>
<tif>GTC</tif>
<ocagroupid>1234</ocagroupid>
</signal>
</child>
</signal>
</collective2>
Essentially, in the response above, C2 is
telling you that signal id 26618011 is an order to BTO 100
shares of AAPL at 10.25 limit. There are two conditional
orders (children) attached to it. They are signals 26618014
and 26618016, and they are respectively a profit target of
99.99 (limit order) and stop loss of 7.5. Both conditionals
are GTC.
Update:
October 31, 2007
When you request signalstatus and
showdetails=1, C2 will return a field called <ocagroupid>xxx</ocagroupid>.
xxx is the OCA (one-cancels-all) group id which the signal
is a part of. If the signal is not part of a still-valid OCA
group, xxx will be blank.
The command called positionstatus
allows you to request the up-to-date status of a C2 position
for any given symbol. This is not meant to be used for
AutoTrading, since it displays only the C2 "model
account's" position. There is no reference to any
real-life brokerage account, nor any scaling calculations.
For true AutoTrade development, be sure you look at the C2ATI
instead of the Trade Placement API (see below).
To use the positionstatus command, you need
to specify a systemid and a symbol:
Responses look like this:
<collective2>
<status>OK</status>
<positionstatus>
<calctime>2006-09-11 10:40:35:000</calctime>
<symbol>EURUSD</symbol>
<position>4</position>
</positionstatus>
</collective2>
In the <position> field, a positive
number means a long position, and negative means a short
position.
The command allsystems returns
a list of the trading systems "owned" by a
particular C2 user. Please note that the list returns only
the trading systems started by the customer -- not the
systems subscribed to by the customer.
To use the allsystems command, you need
to specify C2 user login information like this:
Responses look like this:
<collective2>
<status>ok</status>
<errortype></errortype>
<comment></comment>
<systemsowned>
<system>
<systemid>13262198</systemid>
<systemname>X
System</systemname>
</system>
<system>
<systemid>11138919</systemid>
<systemname>Velocity
X</systemname>
</system>
<system>
<systemid>19308448</systemid>
<systemname>Lotsa
Forex</systemname>
</system>
<system>
<systemid>19472734</systemid>
<systemname>Forex
Tiger</systemname>
</system>
<system>
<systemid>22892798</systemid>
<systemname>Raptor
FX</systemname>
</system>
</systemsowned>
</collective2>
If no systems are "owned" by the user in question,
an error is returned like this:
<collective2>
<status>error</status>
<errortype>No systems owned</errortype>
<comment></comment>
</collective2>
The command called getsystemhypothetical
(getsystemhypo
also accepted) allows you to request equity information
about trading systems on Collective2. Important: the data
returned reveals Collective2's hypothetical
system account information. The data does not include any
customer/brokerage real-life account data.
Here is an example function call:
You can request between one and 25 systems at a time.
Demarcate each systemid using the period (.), as the example
above shows.
Data is returned as in the format below. Most likely, you
will be interested only in the 'totalequityavail' field.
You'll notice that totalequityavail equals cash plus
equity minus marginused.
<collective2>
<hypotheticalEquity>
<system>
<systemid>13889808</systemid>
<systemid>Absolute
Returns</systemid>
<totalequityavail>48281.90</totalequityavail>
<cash>101864.90</cash>
<equity>-8863</equity>
<marginused>44720</marginused>
</system>
<system>
<systemid>13202557</systemid>
<systemid>extreme-os</systemid>
<totalequityavail>218505.23</totalequityavail>
<cash>226803.23</cash>
<equity>894200</equity>
<marginused>902498</marginused>
</system>
</hypotheticalEquity>
</collective2>
The command getallsignals returns
a list of all signalids which are pending, for all systems
to which a user has access. This command was originally
found in the C2ATI (and is still available through that
separate API) but at least one developer requested that the
same command also be made available here in the order
placement API.
The command simply returns a list of
signalids, organized by system. All signalsids are pending
-- that is, they have neither been filled, canceled, nor
expired.
To use the getallsignals command, you need
to specify C2 user login information like this:
Responses look like this:
<collective2>
<status>OK</status>
<allPendingSignals>
<system>
<systemid>13262198</systemid>
<pendingblock>
</pendingblock>
</system>
<system>
<systemid>11138919</systemid>
<pendingblock>
</pendingblock>
</system>
<system>
<systemid>12627477</systemid>
<pendingblock>
<signalid>26123758</signalid>
</pendingblock>
</system>
<system>
<systemid>12646748</systemid>
<pendingblock>
</pendingblock>
</system>
<system>
<systemid>15816213</systemid>
<pendingblock>
<signalid>26990089</signalid>
<signalid>27176705</signalid>
<signalid>27176730</signalid>
<signalid>27192490</signalid>
<signalid>27229357</signalid>
<signalid>27229363</signalid>
<signalid>27271727</signalid>
<signalid>27431975</signalid>
<signalid>27567130</signalid>
<signalid>27621823</signalid>
<signalid>27621827</signalid>
<signalid>27621830</signalid>
<signalid>27621833</signalid>
<signalid>27621836</signalid>
<signalid>27621839</signalid>
<signalid>27621842</signalid>
<signalid>27621845</signalid>
<signalid>27621848</signalid>
<signalid>27621851</signalid>
<signalid>27621856</signalid>
<signalid>27621859</signalid>
<signalid>27621945</signalid>
<signalid>27621949</signalid>
<signalid>27621952</signalid>
<signalid>27621955</signalid>
<signalid>27621959</signalid>
<signalid>27621963</signalid>
</pendingblock>
</system>
</allPendingSignals>
</collective2>
The command addtoocagroup will add an
already-existing signal into an already-existing ocagroup.
This function is not meant to be used to create a brand new
ocagroup.
To use the addtoocagroup command, you need
to specify C2 user login information like this:
Responses look like this:
<collective2>
<status>OK</status>
<details>Signal 12345 now added to ocagroup
9876</details>
</collective2>
The newcomment
command (using the signal comment field to store
information)
Some C2 software developers have
begun to use the <comment> field to store internal
program state information. This wasn't what the field was
originally intended for, but it is ingenious. To aid these
efforts, we have added a new API command called newcomment.
You call it with a particular signalid and it overwrites the
commentary field for that signal. Only system owners have
permission to do this, obviously. You should be aware that
once a signal has been emailed to subscribers, if you change
the comment field, email subscribers will NOT receive the
new commentary, although it will be visible to ITM
subscribers or to anyone visiting the system page on the C2
Web site who turns on "show comments." Given that
the newcomment command is really meant only to store
internal state information for your application, this is not
necessarily a limitation. Here's an example of how to use
the newcomment field:
(Comment text must be URL
encoded.)
<collective2>
<status>OK: Signal
29148580 comment created</status>
<signalid> 29148580</signalid>
<previousComment></previousComment>
</collective2>
The previousComment field will
contain the comment text you are overwriting. This will be
URL Encoded.
Example
of using various API calls
Here is an example of how you
can submit orders, get information about them, and use the
comment field to store information internal to your
application.
First, we place an entry order
with an associated stop loss and profit target.
Response:
<collective2>
<signalid>29148577</signalid>
<comments>Signal 29148577 accepted for
immediate processing.</comments>
<oca></oca>
<delay></delay>
<profittargetsignalid>29148580</profittargetsignalid>
<stoplosssignalid>29148580</stoplosssignalid>
<autoOCAgroupid>29148580</autoOCAgroupid>
</collective2>
Note that the signal IDs have
been assigned for us. Since there are 2 children orders
(stop loss and profit target), they are made into an OCA
group (one cancels another). This means that if either is
filled or cancelled, the other order will be canceled and
will not be left "hanging." If you prefer to
manage you own OCA groups, you can prevent an autoOCAgroupID
from being assigned by adding a &forcenooca=1
parameter to the cmd=signal call. This may be preferable
because it will allow you to change stop losses (by
canceling the stop loss and then submitting a new order)
without affecting the profit-target order, or vice-versa.
Now let's look at the signals
that are pending for a particular user. The getallsignals
command will return the signals that are pending for a given
C2 customer. If I am subscribed to 10 systems, when I send
my email/password combination, I will receive a list of 20
nodes, one for each system. System developers/owners are
treated automatically as subscribers to their own systems,
even if they do not formally subscribe on C2.
http://www.collective2.com/cgi-perl/signal.mpl?c2email=MYEMAIL&pw=PASSWORD&cmd=getallsignals
<collective2>
<status>OK</status>
<allPendingSignals>
<system>
<systemid>13889808</systemid>
<pendingblock>
<signalid>29148580</signalid>
<signalid>29148582</signalid>
<signalid>29148577</signalid>
</pendingblock>
</system>
</allPendingSignals>
</collective2>
One can imagine writing an app
which will cycle through the appropriate signalids above,
requesting more information using the signalstatus
command. Here is an example request.
<collective2>
<signal>
<signalid>29148580</signalid>
<systemname>Absolute Returns</systemname>
<postedwhen>2007-11-13 09:36:18:000</postedwhen>
<emailedwhen>2007-11-13 09:36:55:000</emailedwhen>
<killedwhen>0</killedwhen>
<expiredwhen>0</expiredwhen>
<tradedwhen>0</tradedwhen>
<tradeprice>0</tradeprice>
<action>STC</action>
<quant>10</quant>
<symbol>@ESZ7</symbol>
<limit>1400</limit>
<stop>0</stop>
<market>0</market>
<tif>GTC</tif>
<ocagroupid>29148579</ocagroupid>
<comment></comment>
</signal>
</collective2>
The command called minbuypower lets you set via the API the same setting that you can control via the Edit System Details screen when you are the administrator of a trading system. Here is how we describe this setting on that screen:
Some systems issue a flurry of opening orders at the beginning of the day (that is, orders to enter positions), then wait to see which will get filled, and then cancel the remaining orders when a sufficient amount of "buying power" has been used. We can help you implement this kind of strategy. Below, you can tell C2 to cancel all pending opening orders when your system buying power drops below a certain dollar-value threshold. Orders "to close" positions will not be affected. If you don't understand this paragraph, you probably don't want to use this feature.
Some users have requested that this setting be changeable via the signal API. To meet these requests, we allow you to change this value like this:
Please be aware that, regardless of whether you use the API to set this value or the manual Edit System Details page, this parameter is a best-efforts setting, and C2 cannot guarantee that your buy power level will be calculated and recognized instantly, or that all signals will be canceled speedily. But, overall, the function is pretty reliable, and some system developers do find it useful.
Use the API to send a message to all your subscribers. From the subscribers' perspective, this will appear exactly as it would if you used the Subscriber Broadcast feature available from your system page. Messages need to be short enough to fit in a single URL address, and must be URL Encoded. An example of this function's use:
http://www.collective2.com/cgi-perl/signal.mpl?cmd=sendSubscriberBroadcast&systemid=1234&pw=C2_Password &c2email=Your_C2_login_email&message=The%20S%26P%20is%20a%20useful %20instrument%20%3Cb%3Ebut%3C%2Fb%3E%20only
%20somewhat%20So%20%22Forget%22%20it.
Requests the total C2 Model Account equity of a system. This will return the most recent data point on the system equity chart. Or, more technically speaking, it will return the value of this equation: cash + value of positions currently held (marked-to-market).
http://www.collective2.com/cgi-perl/signal.mpl?cmd=getsystemequity&systemid=1234&pw=C2_Password &c2email=Your_C2_login_email
For stock orders only, you may choose to submit your order quantity by dollar value instead of number of shares. Instead of using &quant= you may use &dollars=.
Note that prices may be delayed, and that the last price at the time of the order submission will be used. In other words, if you submit an order while the market
is closed, the previous day's last price will be used to translate the dollar value you specify into the number of shares. The next day's opening price will not be used.
For stock orders only, you may choose to submit your order quantity as a percentage of account value. Instead of using &quant= you may use &accountpercent=.
Use a number between 1 and 100. For example, the parameter &accountpercent=50 means you want to use 50% of your account value. The account value used (essentially, the most recent Y-axis value of your system equity chart) may be delayed. Then, once that account value number is determined, it is translated into a dollar-value of the amount of shares you want to buy/sell. At this point, the same limitations described n the paragraph above will apply: the last price at the time of the order submission will be used.
Also available: C2 AutoTrading API (C2ATI)
As you can see, the API described on this page describes
how to place trades and trading-related instructions into
C2. If you are interested in pulling trading information out
of C2 (for example, if you want to write software which
interfaces to a broker and then places certain
C2-recommended trades automatically), please contact Collective Help Desk
and ask for more information on the C2 AutoTrading
Interface.
Also available: C2 Data Services API (C2DS)
If you are interested in automating analysis of or interaction with C2 Trading Systems, you should look at our C2 Data Services API.
|